本系列代码托管于:https://github.com/chintsan-code/opencv4-tutorials
本篇使用的项目为:findContours
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main(int argc, const char** argv) {
Mat src = imread("../sample/morph3.png");
if (src.empty()) {
cout << "could not load image..." << endl;
return -1;
}
namedWindow("input", WINDOW_AUTOSIZE);
imshow("input", src);
// 二值化
GaussianBlur(src, src, Size(3, 3), 0);
Mat gray, binary;
cvtColor(src, gray, COLOR_BGR2GRAY);
threshold(gray, binary, 0, 255, THRESH_BINARY | THRESH_OTSU);
// 轮廓发现
imshow("binary", binary);
vector<vector<Point>> contours;
vector<Vec4i> hirearchy;
findContours(binary, contours, hirearchy, RETR_TREE, CHAIN_APPROX_SIMPLE, Point());
//drawContours(src, contours, -1, Scalar(0, 0, 255), 2, 8);
RNG rng(123456);
for (size_t t = 0; t < contours.size(); t++) {
Scalar color = Scalar(rng.uniform(0, 256), rng.uniform(0, 256), rng.uniform(0, 256));
drawContours(src, contours, t, color, 2, 8);
}
imshow("find contours demo", src);
waitKey(0);
destroyAllWindows();
return 0;
}
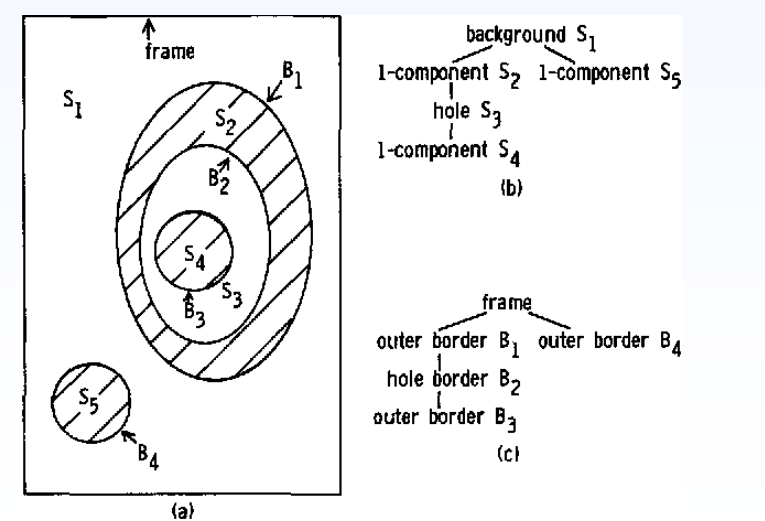
findContours:发现轮廓
void findContours( InputArray image, OutputArrayOfArrays contours, OutputArray hierarchy, int mode, int method, Point offset = Point());
- image:输入图像,8-bit单通道。一般输入二值图。如果不是二值图,会将非零像素视为1,0像素保持0,因此也就相当于二值图输入
- contours:输出轮廓。每个轮廓都是点的集合,一张图像中又有多个轮廓,因此可以用
std::vector<std::vector<cv::point>>
来存储图像中的所有轮廓 - hierarchy:层次结构的拓扑信息。
std::vector<cv::Vec4i>
- mode:轮廓发现算法
- RETR_EXTERNAL:找最大外接轮廓
- RETR_LIST
- RETR_TREE
- method:轮廓编码方式
- CHAIN_APPROX_NONE
- CHAIN_APPROX_SIMPLE
- offset:轮廓的可选偏移量
drawContours:画轮廓
drawContours( InputOutputArray image, InputArrayOfArrays contours, int contourIdx, const Scalar& color, int thickness = 1, int lineType = LINE_8, InputArray hierarchy = noArray(), int maxLevel = INT_MAX, Point offset = Point() );
- image:绘制的背景,在image上绘制轮廓
- contours:需要绘制的轮廓集合
- contourIdx:想要绘制的轮廓contours的索引index。如果要绘制所有轮廓,则输入负数
- color:颜色
- thickness:线宽
- lineType:绘制时的渲染方式
- LINE_4
- LINE_8
- LINE_AA:反锯齿。会更加平滑
- hierarchy:可选参数
- maxLevel:可选参数
- offset:可选参数
评论 (0)